A Python Dictionary is a data structure composed of keys and values, and it's used in various data processing tasks. In this post, we will introduce various methods to extract keys and values from a Dictionary separately and convert them into lists.
1. Extracting Only Keys from a Dictionary
To extract keys from a Dictionary, use the keys()
method. This method returns all keys of the Dictionary, and you can wrap it with list()
to convert it into a list.
Example 1: Using the keys()
Method
my_dict = {'name': 'Alice', 'age': 25, 'city': 'Seoul'}
# Extracting keys into a list
keys_list = list(my_dict.keys())
print(keys_list) # Output: ['name', 'age', 'city']
Example 2: Using List Comprehension
You can also extract keys into a list using the keys()
method along with a for
loop.
keys_list = [key for key in my_dict]
print(keys_list) # Output: ['name', 'age', 'city']
2. Extracting Only Values from a Dictionary
To extract values from a Dictionary, use the values()
method. This method returns all values of the Dictionary, and you can similarly convert it using list()
.
Example 1: Using the values()
Method
my_dict = {'name': 'Alice', 'age': 25, 'city': 'Seoul'}
# Extracting values into a list
values_list = list(my_dict.values())
print(values_list) # Output: ['Alice', 25, 'Seoul']
Example 2: Using List Comprehension
You can also extract values from the Dictionary into a list using a for
loop.
values_list = [value for value in my_dict.values()]
print(values_list) # Output: ['Alice', 25, 'Seoul']
3. Extracting Keys and Values Simultaneously
If you want to extract keys and values into their respective lists at the same time, you can use the following methods.
Example: Using keys()
and values()
Together
my_dict = {'name': 'Alice', 'age': 25, 'city': 'Seoul'}
# Extracting keys and values into lists simultaneously
keys_list = list(my_dict.keys())
values_list = list(my_dict.values())
print(keys_list) # Output: ['name', 'age', 'city']
print(values_list) # Output: ['Alice', 25, 'Seoul']
Example: Using items()
and Unpacking
my_dict = {'name': 'Alice', 'age': 25, 'city': 'Seoul'}
keys_list = [key for key, _ in my_dict.items()]
values_list = [value for _, value in my_dict.items()]
print(keys_list) # Output: ['name', 'age', 'city']
print(values_list) # Output: ['Alice', 25, 'Seoul']
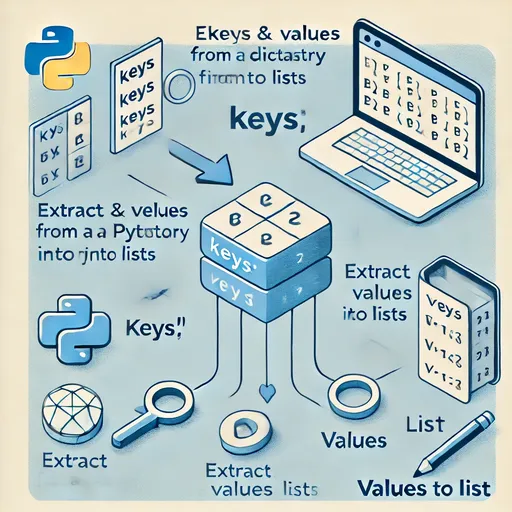
4. Creating a List of Combined Keys and Values
If you want to store keys and values from a Dictionary as pairs in a list, you can use the items()
method.
Example: Creating a List of Key-Value Pairs
my_dict = {'name': 'Alice', 'age': 25, 'city': 'Seoul'}
# Extracting key-value pairs into a list
pairs_list = list(my_dict.items())
print(pairs_list) # Output: [('name', 'Alice'), ('age', 25), ('city', 'Seoul')]
5. Application: Creating a Sorted List of Keys or Values
If you want to create sorted lists of keys and values, you can use sorted()
.
Example 1: Sorting Keys into a List
my_dict = {'b': 2, 'a': 1, 'c': 3}
sorted_keys = sorted(my_dict.keys())
print(sorted_keys) # Output: ['a', 'b', 'c']
Example 2: Sorting Values into a List
sorted_values = sorted(my_dict.values())
print(sorted_values) # Output: [1, 2, 3]
6. Summary
Operation | Method | Example Code |
---|---|---|
Extract only keys into a list | list(my_dict.keys()) |
keys_list = list(my_dict.keys()) |
Extract only values into a list | list(my_dict.values()) |
values_list = list(my_dict.values()) |
Extract key-value pairs into a list | list(my_dict.items()) |
pairs_list = list(my_dict.items()) |
Create a sorted list of keys | sorted(my_dict.keys()) |
sorted_keys = sorted(my_dict.keys()) |
Create a sorted list of values | sorted(my_dict.values()) |
sorted_values = sorted(my_dict.values()) |
Utilize the various methods to extract keys and values from a Python Dictionary into lists for more efficient data processing tasks! 😊
Add a New Comment