Django中發送HTML電子郵件的 render_to_string
徹底分析
在進行Django開發時,經常會使用 django.core.mail.send_mail
發送電子郵件。如果只是發送簡單的純文本電子郵件,則問題不大,但當想要發送更具吸引力和功能性的電子郵件時,就需要HTML格式。此時,可以有效利用的方法就是 render_to_string
。今天我們將徹底分析這個 render_to_string
並探討它在電子郵件發送中的應用。
render_to_string
方法是什麼?
render_to_string
是Django提供的模板渲染方法,可以根據HTML模板文件生成字符串。通過這個方法,可以動態生成模板文件中的HTML內容,並將其用於電子郵件或PDF等各種目的。
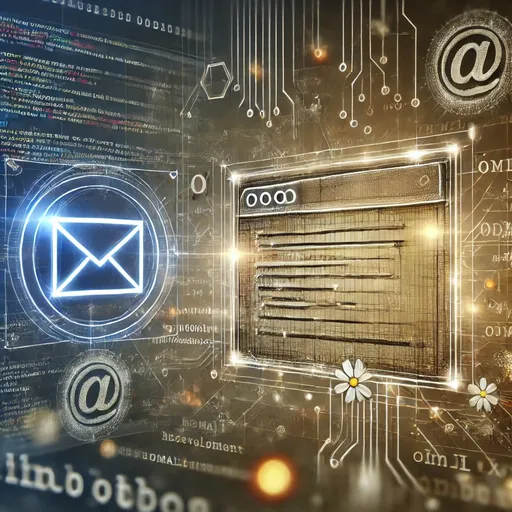
render_to_string
的基本用法
render_to_string
的使用格式為:
from django.template.loader import render_to_string
html_content = render_to_string('template_name.html', context)
主要參數:
template_name
: 要渲染的模板文件的路徑context
: 在模板中使用的數據(字典形式)request
(可選):渲染模板時使用的請求對象
示例 1: 發送HTML電子郵件
我們來看一下如何使用 send_mail
和 render_to_string
發送HTML電子郵件。
模板文件 (templates/emails/welcome_email.html
)
<!DOCTYPE html>
<html>
<head>
<title>歡迎郵件</title>
</head>
<body>
<h1>你好,{{ username }}!</h1>
<p>歡迎使用我們的服務。請單擊以下鏈接以驗證您的帳戶:</p>
<a href="{{ verification_link }}">驗證您的帳戶</a>
</body>
</html>
Python 代碼
from django.core.mail import send_mail
from django.template.loader import render_to_string
# 定義數據
context = {
'username': 'John Doe',
'verification_link': 'https://example.com/verify?token=abc123'
}
# 渲染模板
html_content = render_to_string('emails/welcome_email.html', context)
# 發送電子郵件
send_mail(
subject="歡迎來到我們的服務!",
message="這是一條純文本的後備消息。",
from_email="your_email@example.com",
recipient_list=["recipient@example.com"],
html_message=html_content
)
在這段代碼中,我們使用 render_to_string
將HTML模板轉換為字符串,並將其傳遞給 html_message
參數以發送HTML電子郵件。
示例 2: 與 request
對象一起使用
render_to_string
不僅僅是渲染HTML,還可以直接傳遞request
對象。通過這樣做,可以在模板中利用上下文處理器提供的數據,如登錄用戶信息。
模板文件 (templates/emails/user_info_email.html
)
<!DOCTYPE html>
<html>
<head>
<title>用戶信息</title>
</head>
<body>
<h1>你好,{{ user.username }}!</h1>
<p>您的電子郵件:{{ user.email }}</p>
</body>
</html>
Python 代碼
from django.template.loader import render_to_string
html_content = render_to_string('emails/user_info_email.html', {}, request=request)
# 確認結果
print(html_content)
使用時注意事項
- 傳遞上下文數據:模板中使用的所有變量必須包含在上下文中。
- 檢查模板路徑:模板文件必須準確地位於指定的目錄中。
- HTML驗證:檢查生成的HTML在瀏覽器或電子郵件客戶端中,以確保樣式不會被破壞。
總結
render_to_string
可以將模板文件渲染為字符串,在Django中非常有用。特別是可以直接傳遞request
對象,因此能夠輕鬆使用動態數據。在實現將電子郵件以HTML格式發送的代碼時,務必參考這個方法! 😊
目前沒有評論。