Django Forms的核心是 字段(Field) 和 小工具(Widget)。字段負責驗證和處理輸入數據,而小工具則定義該字段的HTML元素渲染。在本文中,我們將詳細探討主要字段和小工具及其自定義方法。
1. Django Forms 字段
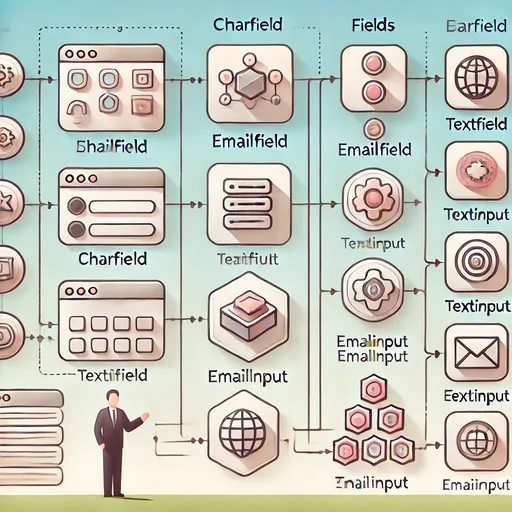
1.1 字段是什麼?
字段是Django Forms中定義和驗證數據的基本組件。
- 在將用戶輸入的數據存儲到
cleaned_data
之前進行驗證。 - 每個字段與HTML輸入類型相關映射。
主要字段類型
字段 | 描述 | 示例小工具 |
---|---|---|
CharField |
字符串輸入字段 | TextInput |
EmailField |
電子郵件格式輸入字段 | EmailInput |
IntegerField |
整數輸入字段 | NumberInput |
DateField |
日期輸入字段 | DateInput |
ChoiceField |
選擇性字段 | Select |
BooleanField |
復選框輸入字段 | CheckboxInput |
FileField |
文件上傳字段 | FileInput |
字段基本用法
from django import forms
class ExampleForm(forms.Form):
name = forms.CharField(max_length=50, required=True, label="您的姓名")
email = forms.EmailField(required=True, label="您的電子郵件")
age = forms.IntegerField(required=False, min_value=1, label="您的年齡")
2. Django Forms 小工具
2.1 小工具是什麼?
小工具是Django Forms中,每個字段在HTML中渲染時使用的元素。
- 定義字段的HTML標籤和屬性。
- 例如:
CharField
預設使用TextInput
小工具。
主要小工具類型
小工具 | 渲染的HTML標籤 | 使用字段 |
---|---|---|
TextInput |
<input type="text"> |
CharField |
EmailInput |
<input type="email"> |
EmailField |
NumberInput |
<input type="number"> |
IntegerField |
DateInput |
<input type="date"> |
DateField |
Select |
<select> |
ChoiceField |
CheckboxInput |
<input type="checkbox"> |
BooleanField |
FileInput |
<input type="file"> |
FileField |
2.2 字段和ID屬性
Django Forms在渲染為HTML時,自動為每個字段添加唯一的 id
屬性。該屬性的格式為 id_<fieldname>
。
- 例如:字段名稱為
name
時,渲染的<input>
標籤的id
為id_name
。 - 該
id
屬性在模板中特別有用:- JavaScript:選擇特定字段以進行動態處理。
- 標籤:通過
<label for="id_name">
進行連結。
示例:字段和ID屬性的使用
<form method="post">
{% csrf_token %}
<label for="id_name">姓名:</label>
<input type="text" id="id_name" name="name">
<script>
document.getElementById('id_name').addEventListener('input', function() {
console.log(this.value);
});
</script>
</form>
3. 字段和小工具的自定義
3.1 字段屬性自定義
Django Forms使用 attrs
更改HTML元素的屬性。
示例:變更字段屬性
class CustomForm(forms.Form):
username = forms.CharField(
max_length=100,
widget=forms.TextInput(attrs={
'class': 'form-control',
'placeholder': '輸入您的用户名'
})
)
email = forms.EmailField(
widget=forms.EmailInput(attrs={
'class': 'form-control',
'placeholder': '輸入您的電子郵件'
})
)
3.2 創建自定義小工具
根據需求可以創建自定義小工具。
示例:創建自定義小工具
from django.forms.widgets import TextInput
class CustomTextInput(TextInput):
template_name = 'custom_widgets/custom_text_input.html'
class CustomForm(forms.Form):
custom_field = forms.CharField(widget=CustomTextInput())
4. 實戰案例:創建樣式化表單
4.1 應用Bootstrap
與Bootstrap一起使用時,可以為每個字段添加 class='form-control'
以進行樣式設計。
示例:Bootstrap樣式表單
class BootstrapForm(forms.Form):
name = forms.CharField(
widget=forms.TextInput(attrs={'class': 'form-control', 'placeholder': '輸入您的姓名'})
)
email = forms.EmailField(
widget=forms.EmailInput(attrs={'class': 'form-control', 'placeholder': '輸入您的電子郵件'})
)
4.2 自定義CSS和表單樣式
可以添加CSS文件以進一步調整表單的樣式。
示例:與CSS相關的模板
<form method="post">
{% csrf_token %}
<div class="form-group">
<label for="id_name">您的姓名</label>
<input type="text" class="form-control" id="id_name" name="name">
</div>
<div class="form-group">
<label for="id_email">您的電子郵件</label>
<input type="email" class="form-control" id="id_email" name="email">
</div>
<button type="submit" class="btn btn-primary">提交</button>
</form>
5. 結論
Django Forms的字段和小工具在處理和驗證數據以及構建用戶界面方面發揮了核心作用。除了基本提供的字段和小工具外,還可以根據需要進行自定義設計以適應項目。
在下一篇文章中,我們將更深入探討 驗證和表單自定義。
目前沒有評論。