Django에서 HTML 이메일을 보내기 위한 render_to_string
철저 분석
Django 개발을 하다 보면 django.core.mail.send_mail
을 사용해 이메일을 보내는 일이 종종 있습니다. 단순한 텍스트 이메일만 보내는 경우라면 문제가 없지만, 보다 매력적이고 기능적인 이메일을 보내고자 할 때는 HTML 형식이 필요합니다. 이때 유용하게 활용할 수 있는 메서드가 바로 render_to_string
입니다. 오늘은 이 render_to_string
을 철저하게 분석해보고, 이메일 전송에 활용하는 방법을 살펴보겠습니다.
render_to_string
메서드란?
render_to_string
은 Django에서 제공하는 템플릿 렌더링 메서드로, HTML 템플릿 파일을 기반으로 문자열을 생성할 수 있습니다. 이를 통해 템플릿 파일에서 동적으로 HTML 콘텐츠를 생성하고, 이를 이메일이나 PDF와 같은 다양한 목적으로 사용할 수 있습니다.
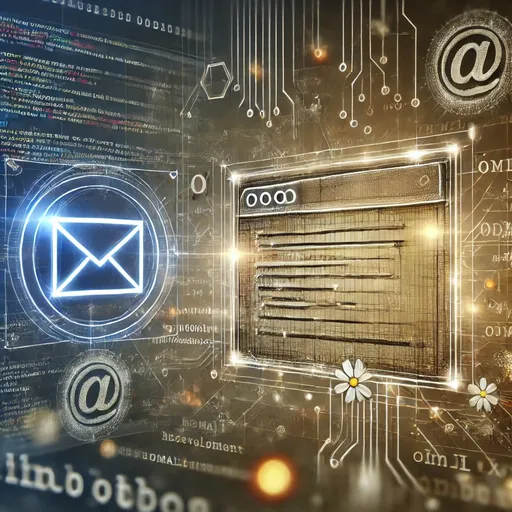
render_to_string
기본 사용법
render_to_string
은 다음과 같은 형식으로 사용됩니다:
from django.template.loader import render_to_string
html_content = render_to_string('template_name.html', context)
주요 매개변수:
template_name
: 렌더링할 템플릿 파일의 경로context
: 템플릿에서 사용할 데이터(딕셔너리 형태)request
(선택): 템플릿 렌더링 시 사용할 요청 객체
예제 1: HTML 이메일 전송
HTML 이메일을 전송하기 위해 send_mail
과 함께 render_to_string
을 사용하는 방법을 살펴보겠습니다.
템플릿 파일 (templates/emails/welcome_email.html
)
<!DOCTYPE html>
<html>
<head>
<title>Welcome Email</title>
</head>
<body>
<h1>Hello, {{ username }}!</h1>
<p>Welcome to our service. Please click the link below to verify your account:</p>
<a href="{{ verification_link }}">Verify Your Account</a>
</body>
</html>
Python 코드
from django.core.mail import send_mail
from django.template.loader import render_to_string
# 데이터 정의
context = {
'username': 'John Doe',
'verification_link': 'https://example.com/verify?token=abc123'
}
# 템플릿 렌더링
html_content = render_to_string('emails/welcome_email.html', context)
# 이메일 전송
send_mail(
subject="Welcome to Our Service!",
message="This is a plain text fallback message.",
from_email="your_email@example.com",
recipient_list=["recipient@example.com"],
html_message=html_content
)
이 코드에서는 render_to_string
을 사용하여 HTML 템플릿을 문자열로 변환하고, 이를 html_message
매개변수에 전달하여 HTML 이메일을 보냈습니다.
예제 2: request
객체와 함께 사용
render_to_string
은 단순히 HTML만 렌더링하는 것이 아니라, request
객체를 직접 전달할 수도 있습니다. 이를 통해 로그인된 사용자 정보 등 컨텍스트 프로세서에서 제공하는 데이터를 템플릿에서 활용할 수 있습니다.
템플릿 파일 (templates/emails/user_info_email.html
)
<!DOCTYPE html>
<html>
<head>
<title>User Info</title>
</head>
<body>
<h1>Hello, {{ user.username }}!</h1>
<p>Your email: {{ user.email }}</p>
</body>
</html>
Python 코드
from django.template.loader import render_to_string
html_content = render_to_string('emails/user_info_email.html', {}, request=request)
# 결과 확인
print(html_content)
사용 시 주의사항
- 컨텍스트 데이터 전달: 템플릿에서 사용하는 모든 변수는 컨텍스트에 포함되어야 합니다.
- 템플릿 경로 확인: 템플릿 파일은 지정된 디렉토리에 정확히 위치해야 합니다.
- HTML 검증: 생성된 HTML을 브라우저나 이메일 클라이언트에서 확인하여 스타일이 깨지지 않도록 검증하세요.
마무리
render_to_string
은 템플릿 파일을 문자열로 렌더링할 수 있어 Django에서 매우 유용하게 사용됩니다. 특히 request
객체를 직접 전달할 수 있으므로, 동적 데이터를 쉽게 활용할 수 있습니다. 이메일을 HTML 형식으로 보내는 코드를 구현할 때 꼭 사용해 보세요! 😊
Add a New Comment