DjangoでHTMLメールを送信するための render_to_string
徹底分析
Django開発をしていると、django.core.mail.send_mail
を使ってメールを送信することがしばしばあります。単純なテキストメールのみを送信する場合は問題ありませんが、より魅力的で機能的なメールを送りたい場合はHTML形式が必要です。この時に便利に活用できるメソッドがrender_to_string
です。今日はこのrender_to_string
を徹底的に分析し、メール送信に活用する方法を見ていきましょう。
render_to_string
メソッドとは?
render_to_string
はDjangoが提供するテンプレートレンダリングメソッドで、HTMLテンプレートファイルを基に文字列を生成することができます。これにより、テンプレートファイルから動的にHTMLコンテンツを生成し、これをメールやPDFなどの様々な目的で使用できます。
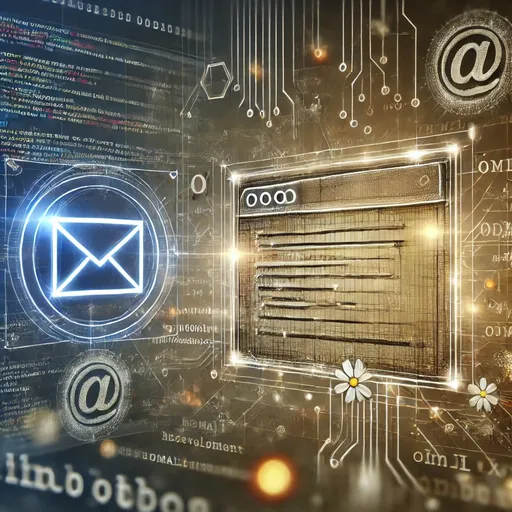
render_to_string
基本使用法
render_to_string
は次のような形式で使用されます:
from django.template.loader import render_to_string
html_content = render_to_string('template_name.html', context)
主なパラメータ:
template_name
: レンダリングするテンプレートファイルのパスcontext
: テンプレートで使用するデータ(辞書形式)request
(任意): テンプレートレンダリング時に使用するリクエストオブジェクト
例1: HTMLメール送信
HTMLメールを送信するためにsend_mail
と共にrender_to_string
を使用する方法を見ていきます。
テンプレートファイル (templates/emails/welcome_email.html
)
<!DOCTYPE html>
<html>
<head>
<title>Welcome Email</title>
</head>
<body>
<h1>Hello, {{ username }}!</h1>
<p>Welcome to our service. Please click the link below to verify your account:</p>
<a href="{{ verification_link }}">Verify Your Account</a>
</body>
</html>
Pythonコード
from django.core.mail import send_mail
from django.template.loader import render_to_string
# データ定義
context = {
'username': 'John Doe',
'verification_link': 'https://example.com/verify?token=abc123'
}
# テンプレートレンダリング
html_content = render_to_string('emails/welcome_email.html', context)
# メール送信
send_mail(
subject="Welcome to Our Service!",
message="This is a plain text fallback message.",
from_email="your_email@example.com",
recipient_list=["recipient@example.com"],
html_message=html_content
)
このコードではrender_to_string
を使ってHTMLテンプレートを文字列に変換し、これをhtml_message
パラメータに渡してHTMLメールを送信しました。
例2: request
オブジェクトと共に使用
render_to_string
は単にHTMLをレンダリングするだけでなく、request
オブジェクトを直接渡すことも可能です。これにより、ログインしているユーザー情報などコンテキストプロセッサで提供されるデータをテンプレート内で活用できます。
テンプレートファイル (templates/emails/user_info_email.html
)
<!DOCTYPE html>
<html>
<head>
<title>User Info</title>
</head>
<body>
<h1>Hello, {{ user.username }}!</h1>
<p>Your email: {{ user.email }}</p>
</body>
</html>
Pythonコード
from django.template.loader import render_to_string
html_content = render_to_string('emails/user_info_email.html', {}, request=request)
# 結果確認
print(html_content)
使用時の注意事項
- コンテキストデータの伝達: テンプレートで使用するすべての変数はコンテキストに含まれている必要があります。
- テンプレートパスの確認: テンプレートファイルは指定されたディレクトリに正確に配置されている必要があります。
- HTML検証: 生成されたHTMLをブラウザやメールクライアントで確認して、スタイルが崩れていないか検証してください。
まとめ
render_to_string
はテンプレートファイルを文字列にレンダリングできるため、Djangoで非常に有用に使用されます。特にrequest
オブジェクトを直接渡すことができるので、動的なデータを簡単に活用できます。HTML形式でメールを送信するコードを実装する際はぜひ試してみてください! 😊
Add a New Comment