Today's exploration topic is When Does the Django request Object Disappear? 🎯
While developing with Django, the request
object appears automatically from somewhere and seems to disappear naturally after a response. However, I suddenly had this thought.
"When exactly does this
request
object get destroyed?""Could it be that it stays in memory forever? If so, do we need to manually clean up the memory?"
"In that case, wouldn't there be a potential for memory leaks if there are many requests?"
To resolve this curiosity, I deeply explored the request handling flow in Django and uncovered the lifespan and memory management of the request
object through actual Django internal code and experiments! 🚀
1️⃣ When Are request Objects Created and Cleared?
Django automatically creates the request object when it receives a user's HTTP request. This means developers can use the request
object directly in the view function without needing to create it themselves.
def my_view(request):
user_ip = request.META.get('REMOTE_ADDR')
return HttpResponse(f"Your IP: {user_ip}")
The request handling in Django proceeds as follows.
Step | Action |
---|---|
1️⃣ | The client sends a request |
2️⃣ | Django creates the request object |
3️⃣ | The request object is passed through middleware to the view function |
4️⃣ | The view function uses the request object to generate a response |
5️⃣ | Once the response is completed, the request object is automatically freed from memory |
📌 In other words, the request
object is created when the request starts, and it is destroyed when the response is completed.
📌 Django automatically sets the request
object to None after the request ends to free up memory.
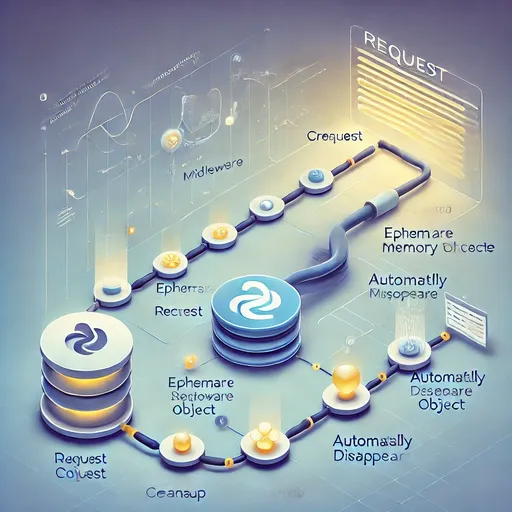
🔍 Checking Django's Internal Code
In the BaseHandler
class (django.core.handlers.base.BaseHandler
) that handles request processing in Django, there is a part where the request
object is automatically cleaned up when the request ends.
def _get_response(self, request):
response = None
try:
response = self.process_request(request) # Creation and processing of the request object
finally:
request = None # Setting the request object to None to free memory
return response
🚀 This means that developers do not need to perform actions like del request
directly
🚀 The garbage collector (GC) in Python detects this and frees the memory.
🎯 Conclusion: Django's request Object is Quite Smart!
🙌 After finishing my research, I realized that the Django request
object is a very useful tool because once created, it is processed quickly, does not consume DB queries, and is destroyed automatically!
📌 In summary:
- Created when an HTTP request starts and automatically deleted when the response is completed.
- Can be utilized as a "cool cache space" to store temporary data for use in views!
- For instance, by using middleware or custom decorator functions,
request.additional_field = some_value
can create a data storage that is valid only for views.- This way, it avoids additional DB queries even if the same data is accessed multiple times in the view,
- and since it gets destroyed automatically after the response, there's no need to worry about memory.
- Performance optimization due to automatic memory management! (Values added to the request object are automatically deleted after the response.)
- Efficient structure that ensures data consistency while serving as a caching mechanism!
🚀 Thus, Django's request
object is an excellent tool that guarantees "automatic memory management + performance optimization + data consistency"! 🎯
🔗 Previous Post: Does request.session.get() Trigger a DB Query?
If you were curious about how the Django request
object is stored and destroyed in memory, make sure to check out the previous discussion on whether request.session.get()
triggers a DB query!
📌 Previous Post: Does Django's request.session.get() Trigger a DB Query?
If you're curious about Django's session management and memory caching, be sure to read on! 🚀
There are no comments.