In-Depth Analysis of render_to_string
for Sending HTML Emails in Django
When developing with Django, it's common to send emails using django.core.mail.send_mail
. If you're only sending simple text emails, there are no issues. However, when you want to send more attractive and functional emails, you'll need HTML format. The method that can be very useful in this case is render_to_string
. Today, we will thoroughly analyze this render_to_string
and explore how to use it for sending emails.
What is the render_to_string
method?
render_to_string
is a template rendering method provided by Django that allows you to generate a string based on an HTML template file. This enables you to dynamically generate HTML content from templates for various purposes like emails or PDFs.
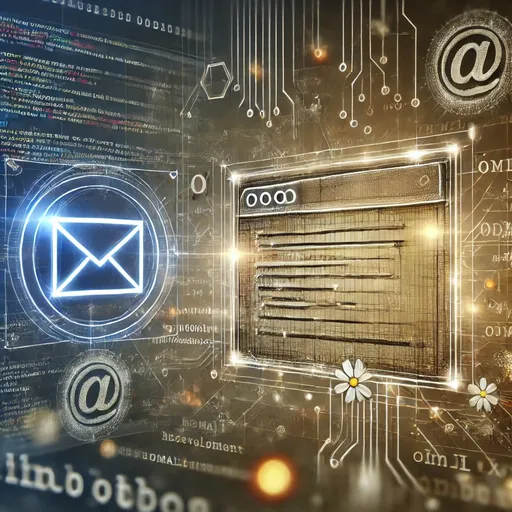
Basic Usage of render_to_string
render_to_string
is used in the following format:
from django.template.loader import render_to_string
html_content = render_to_string('template_name.html', context)
Main Parameters:
template_name
: Path to the template file to be renderedcontext
: Data to be used in the template (in dictionary form)request
(optional): Request object to be used when rendering the template
Example 1: Sending HTML Email
Let's look at how to use send_mail
together with render_to_string
to send an HTML email.
Template File (templates/emails/welcome_email.html
)
<!DOCTYPE html>
<html>
<head>
<title>Welcome Email</title>
</head>
<body>
<h1>Hello, {{ username }}!</h1>
<p>Welcome to our service. Please click the link below to verify your account:</p>
<a href="{{ verification_link }}">Verify Your Account</a>
</body>
</html>
Python Code
from django.core.mail import send_mail
from django.template.loader import render_to_string
# Define data
context = {
'username': 'John Doe',
'verification_link': 'https://example.com/verify?token=abc123'
}
# Render template
html_content = render_to_string('emails/welcome_email.html', context)
# Send email
send_mail(
subject="Welcome to Our Service!",
message="This is a plain text fallback message.",
from_email="your_email@example.com",
recipient_list=["recipient@example.com"],
html_message=html_content
)
In this code, we use render_to_string
to convert the HTML template into a string, which is then passed to the html_message
parameter to send an HTML email.
Example 2: Using with request
object
render_to_string
not only renders HTML but also allows you to directly pass a request
object. This enables you to utilize data provided by a context processor, such as information about logged-in users, in the template.
Template File (templates/emails/user_info_email.html
)
<!DOCTYPE html>
<html>
<head>
<title>User Info</title>
</head>
<body>
<h1>Hello, {{ user.username }}!</h1>
<p>Your email: {{ user.email }}</p>
</body>
</html>
Python Code
from django.template.loader import render_to_string
html_content = render_to_string('emails/user_info_email.html', {}, request=request)
# Check result
print(html_content)
Precautions When Using
- Passing context data: All variables used in the template must be included in the context.
- Template path verification: The template file must be located exactly in the specified directory.
- HTML validation: Check the generated HTML in the browser or email client to ensure the style is not broken.
Conclusion
render_to_string
is extremely useful in Django for rendering template files into strings. Especially, since you can directly pass the request
object, you can easily utilize dynamic data. Be sure to try it out when implementing code to send emails in HTML format! 😊
Add a New Comment