Curiosity About Django's View Functions and Hidden Principles
Developers often write code out of habit. I too used to use request.GET.get('variable')
or request.POST.get('variable')
without any doubt while writing view functions in Django. One day, however, a question suddenly arose in my mind.
"How does this actually work?"
Django's Request Handling: The Secrets of request.GET
and request.POST
To find the answer to this question, I looked into how Django operates. To put it simply, request.GET
and request.POST
are properties of an HTTP request object that make it easier to handle request data sent by the client.
Django parses the data sent by the client to the server and converts it into a form that can be handled like a dictionary. Thus, we can easily retrieve the desired data using request.GET.get('variable')
or request.POST.get('variable')
.
However, the important fact here is that this object is not a real dictionary. So what does the term like a dictionary really mean?
QueryDict: An Object Like a Dictionary, But More Than Just a Dictionary
In Django, request.GET
and request.POST
are actually instances of a class called QueryDict
. This class provides behavior similar to a regular dictionary but has some important differences:
1. Allows Multiple Values for the Same Key
While a regular dictionary can store only one value for a single key, QueryDict
can store multiple values for the same key.
For instance, when you have a query string like ?key=value1&key=value2
:
request.GET.get('key') # 'value1' (the first value)
request.GET.getlist('key') # ['value1', 'value2'] (all values)
2. Immutability
By default, QueryDict
is immutable. To modify or add values, you first need to create a copy:
mutable_querydict = request.GET.copy()
mutable_querydict['new_key'] = 'new_value'
3. More Than Just Dictionary Features
Methods like getlist()
that are not available in regular dictionaries are some of the powerful features provided by QueryDict
.
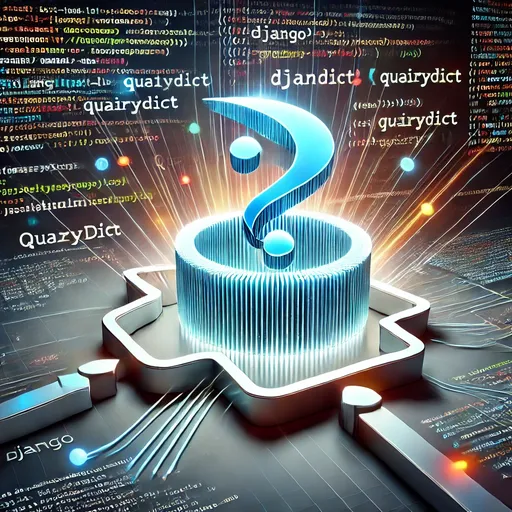
Why Was It Designed This Way?
The reason for this design is simple. In web applications, there is often a need to handle multiple pieces of data with the same name, and maintaining immutability ensures data integrity. QueryDict
meets these requirements while providing a dictionary-style API that developers are familiar with. Users can easily handle complex HTTP request data just like they would with a dictionary.
The Joy of Unraveling Django's Principles
A question sparked by a small curiosity led me to marvel at Django's meticulous and efficient design during the search for answers.
Learning about the existence of QueryDict
and its special features reminded me once again of how developer-friendly the Django framework is.
Try exploring those small curiosities that arise when you use Django. There are surely new delights and inspirations waiting for you. ๐
Happy coding as you explore how Django works! ๐
Add a New Comment