Django provides two main form classes: forms.Form
and forms.ModelForm
. In this article, we will explain the differences between these two classes and when to choose each based on their use cases.
1. Overview of forms.Form
and forms.ModelForm
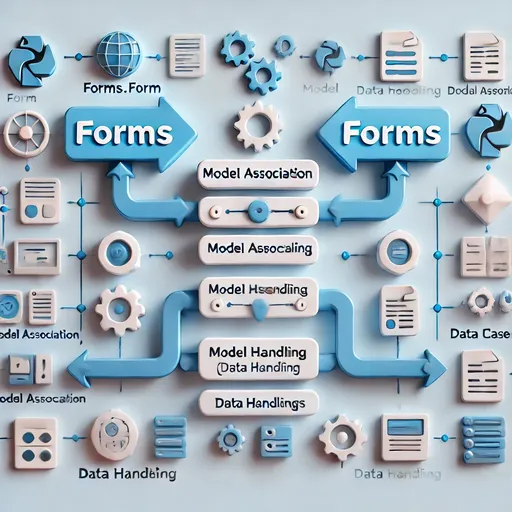
1.1 What is forms.Form
?
- Independent form class: Operates independently of Django models and allows you to manually define the desired data.
- Main usage: Used to simply validate user inputs or handle data that is not directly associated with a model.
Example: User Feedback Form
from django import forms
class FeedbackForm(forms.Form):
name = forms.CharField(max_length=50, label="Your Name")
email = forms.EmailField(label="Your Email")
message = forms.CharField(widget=forms.Textarea, label="Your Feedback")
1.2 What is forms.ModelForm
?
- Form class linked to a model: Directly associated with a Django model, automating interactions with the database.
- Main usage: Used to create or update model instances or to receive data to be saved to the database.
Example: Blog Post Creation Form
from django import forms
from .models import Post
class PostForm(forms.ModelForm):
class Meta:
model = Post
fields = ['title', 'content']
2. Key Differences Comparison
Category | forms.Form | forms.ModelForm |
---|---|---|
Model Association | No association with a model | Directly associated with a model |
Data Handling | Manually save data after validation | Automatically save data (using form.save() method) |
Field Definition | Must define all fields explicitly | Automatically converts model fields to form fields |
Usage | Independent data processing or user input forms | Forms connected to database operations |
Flexibility | Fields can be arranged freely | Dependent on model fields |
3. Use Cases
3.1 Use Cases for forms.Form
- Processing data unrelated to a model: E.g., search forms, login forms, password reset forms.
Example: Search Form
class SearchForm(forms.Form):
query = forms.CharField(max_length=100, label="Search")
- Complex user input handling: E.g., when input fields aren’t directly stored in a model.
Example: Password Change Form
class PasswordChangeForm(forms.Form):
old_password = forms.CharField(widget=forms.PasswordInput, label="Old Password")
new_password = forms.CharField(widget=forms.PasswordInput, label="New Password")
confirm_password = forms.CharField(widget=forms.PasswordInput, label="Confirm Password")
def clean(self):
cleaned_data = super().clean()
new_password = cleaned_data.get("new_password")
confirm_password = cleaned_data.get("confirm_password")
if new_password != confirm_password:
raise forms.ValidationError("Passwords do not match.")
3.2 Use Cases for forms.ModelForm
- Creating and updating model objects: E.g., writing blog posts, submitting comments, editing user profiles.
Example: Comment Submission Form
class CommentForm(forms.ModelForm):
class Meta:
model = Comment
fields = ['author', 'text']
- Input for model-based data: E.g., when modifying existing data or saving to the database.
4. Comparison of Form Operation
4.1 Data Validation
forms.Form
: Defines fields and validation logic manually.forms.ModelForm
: Automatically uses validation rules defined on model fields.
Example: Model Field Validation
class Product(models.Model):
name = models.CharField(max_length=100)
price = models.DecimalField(max_digits=10, decimal_places=2)
class ProductForm(forms.ModelForm):
class Meta:
model = Product
fields = ['name', 'price']
- The
price
field automatically validates constraints defined on the model (max_digits
,decimal_places
).
4.2 Data Saving
forms.Form
: Processes data usingcleaned_data
.forms.ModelForm
: Automatically saves data usingsave()
method.
Saving Data Example with forms.Form
form = FeedbackForm(request.POST)
if form.is_valid():
feedback = Feedback(
name=form.cleaned_data['name'],
email=form.cleaned_data['email'],
message=form.cleaned_data['message']
)
feedback.save()
Saving Data Example with forms.ModelForm
form = PostForm(request.POST)
if form.is_valid():
form.save()
5. Which Form to Choose?
- Tasks related to a model: If you need to interact directly with the database, it is suitable to use
forms.ModelForm
. - Tasks unrelated to a model: If you need independent data validation or user input processing, use
forms.Form
.
Conclusion
forms.Form
and forms.ModelForm
are two powerful tools for handling data input and validation in Django. Since the two classes have different purposes and characteristics, it's important to choose the right form for the situation. In the next article, we will take a closer look at form fields and widgets.
Add a New Comment