The core of Django Forms is the Field and Widget. Fields validate and process input data, while widgets define the HTML elements that render those fields. In this article, we will take a detailed look at the main fields and widgets, as well as how to customize them.
1. Django Forms Fields
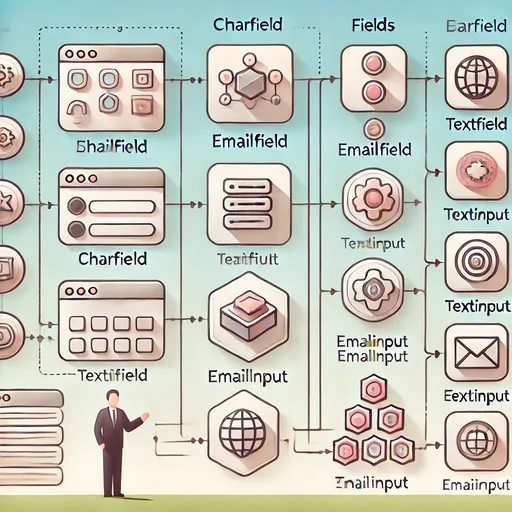
1.1 What is a Field?
A field is a fundamental component that defines and validates data in Django Forms.
- Validates the data input by the user before storing it as
cleaned_data
. - Each field maps to an HTML input type.
Main Types of Fields
Field | Description | Example Widget |
---|---|---|
CharField |
String input field | TextInput |
EmailField |
Email format input field | EmailInput |
IntegerField |
Integer input field | NumberInput |
DateField |
Date input field | DateInput |
ChoiceField |
Option selection field | Select |
BooleanField |
Checkbox input field | CheckboxInput |
FileField |
File upload field | FileInput |
Basic Usage of Fields
from django import forms
class ExampleForm(forms.Form):
name = forms.CharField(max_length=50, required=True, label="Your Name")
email = forms.EmailField(required=True, label="Your Email")
age = forms.IntegerField(required=False, min_value=1, label="Your Age")
2. Django Forms Widgets
2.1 What is a Widget?
A widget is an element used to render each field in HTML within Django Forms.
- Defines the HTML tags and attributes for the field.
- For example,
CharField
typically uses theTextInput
widget by default.
Main Types of Widgets
Widget | Rendered HTML Tag | Used with Field |
---|---|---|
TextInput |
<input type="text"> |
CharField |
EmailInput |
<input type="email"> |
EmailField |
NumberInput |
<input type="number"> |
IntegerField |
DateInput |
<input type="date"> |
DateField |
Select |
<select> |
ChoiceField |
CheckboxInput |
<input type="checkbox"> |
BooleanField |
FileInput |
<input type="file"> |
FileField |
2.2 Field and ID Attributes
Django Forms automatically adds a unique id
attribute to each field when rendered in HTML. This attribute is generated in the id_<fieldname>
format.
- For example, if the field name is
name
, the rendered<input>
tag'sid
will beid_name
. - This
id
attribute is useful in templates in cases such as:- JavaScript: To dynamically handle specific fields.
- Label tags: To connect using
<label for="id_name">
.
Example: Using Fields and ID Attributes
<form method="post">
{% csrf_token %}
<label for="id_name">Name:</label>
<input type="text" id="id_name" name="name">
<script>
document.getElementById('id_name').addEventListener('input', function() {
console.log(this.value);
});
</script>
</form>
3. Customizing Fields and Widgets
3.1 Customizing Field Attributes
Django Forms allows you to modify the attributes of HTML elements using attrs
.
Example: Changing Field Attributes
class CustomForm(forms.Form):
username = forms.CharField(
max_length=100,
widget=forms.TextInput(attrs={
'class': 'form-control',
'placeholder': 'Enter your username'
})
)
email = forms.EmailField(
widget=forms.EmailInput(attrs={
'class': 'form-control',
'placeholder': 'Enter your email'
})
)
3.2 Creating Custom Widgets
You can also create custom widgets as needed.
Example: Creating a Custom Widget
from django.forms.widgets import TextInput
class CustomTextInput(TextInput):
template_name = 'custom_widgets/custom_text_input.html'
class CustomForm(forms.Form):
custom_field = forms.CharField(widget=CustomTextInput())
4. Practical Example: Creating a Styled Form
4.1 Applying Bootstrap
When using Bootstrap, you can add the class='form-control'
to each field for styling.
Example: Bootstrap Styled Form
class BootstrapForm(forms.Form):
name = forms.CharField(
widget=forms.TextInput(attrs={'class': 'form-control', 'placeholder': 'Enter your name'})
)
email = forms.EmailField(
widget=forms.EmailInput(attrs={'class': 'form-control', 'placeholder': 'Enter your email'})
)
4.2 Custom CSS and Form Styling
You can add a CSS file to fine-tune the styling of the form even further.
Example: Template Linked with CSS
<form method="post">
{% csrf_token %}
<div class="form-group">
<label for="id_name">Your Name</label>
<input type="text" class="form-control" id="id_name" name="name">
</div>
<div class="form-group">
<label for="id_email">Your Email</label>
<input type="email" class="form-control" id="id_email" name="email">
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
5. Conclusion
The fields and widgets in Django Forms play a crucial role in processing and validating data and structuring the user interface. In addition to the built-in fields and widgets, you can customize them to design forms that suit your project's needs.
In the next article, we will delve deeper into validation and form customization.
Add a New Comment