Beginners who start learning Python may often confuse JSON format with Python Dictionary. Both formats use {} curly braces and represent data in a key:value structure, but they differ in terms of use and syntax. In this article, we will discuss the similarities, differences, and methods for converting between JSON and Dictionary in Python.
1. Similarities Between JSON and Python Dictionary
Both JSON and Python Dictionary share the commonality of representing data in key-value pairs. This similarity is why beginners often feel they are the same.
Main Similarities
- Key-Value Structure: Both formats store data in a
{key: value}
format. - Support for Nested Structures: They can internally represent data by nesting lists, other objects, etc.
Examples
JSON Format:
{
"name": "Alice",
"age": 25,
"skills": ["Python", "Django"]
}
Python Dictionary:
person = {
"name": "Alice",
"age": 25,
"skills": ["Python", "Django"]
}
2. Differences Between JSON and Python Dictionary
While JSON and Dictionary may initially appear similar, there are differences in syntax and purpose.
2.1 Differences in Purpose
- JSON: A language-independent standard format for data exchange. It is used in various languages such as JavaScript, Java, and C++ for data transmission.
- Python Dictionary: A Python-specific data structure for storing and manipulating data internally within Python.
2.2 Differences in Syntax
JSON has the following syntactical differences compared to Python Dictionary:
Feature | JSON | Python Dictionary |
---|---|---|
String Notation | Strings must be enclosed in " |
Strings can use either ' or " |
Type of Key | Keys must be strings | Keys can be of various types, including strings and numbers |
Type of Value | Limited (strings, numbers, arrays, etc.) | No limitations (can include functions, objects, etc.) |
Boolean Values | true , false |
True , False |
Null Values | null |
None |
Comments | No support for comments | Comments are allowed |
Example of Differences
JSON:
{
"name": "Alice",
"age": 25,
"isActive": true,
"nickname": null
}
Python Dictionary:
person = {
"name": "Alice",
"age": 25,
"isActive": True, # Comment allowed
"nickname": None
}
In the above example:
- In JSON,
true
,false
, andnull
are written in lowercase, but - In Python, they are represented with uppercase initial letters as
True
,False
, andNone
.
3. Converting Between Python Dictionary and JSON
In Python, you can easily convert between JSON and Dictionary using the json
module.
3.1 Converting Dictionary to JSON
You can use json.dumps()
to convert a Python Dictionary into a JSON string.
import json
person = {
"name": "Alice",
"age": 25,
"isActive": True,
"nickname": None
}
json_data = json.dumps(person)
print(json_data)
Output:
{"name": "Alice", "age": 25, "isActive": true, "nickname": null}
3.2 Converting JSON to Dictionary
You can convert a JSON string to a Python Dictionary using json.loads()
.
import json
json_data = '{"name": "Alice", "age": 25, "isActive": true, "nickname": null}'
person = json.loads(json_data)
print(person)
Output:
{'name': 'Alice', 'age': 25, 'isActive': True, 'nickname': None}
3.3 Saving and Reading JSON Data Files
Saving JSON Data to a File
import json
data = {"name": "Alice", "age": 25, "isActive": True}
with open("data.json", "w") as file:
json.dump(data, file, indent=4) # Using indent option
Result Using Indent Option:
- Saved without indent:
{"name": "Alice","age":25,"isActive":true}
- Saved with indent=4:
{ "name": "Alice", "age": 25, "isActive": true }
Reading a JSON File
import json
with open("data.json", "r") as file:
data = json.load(file)
print(data)
Output:
{'name': 'Alice', 'age': 25, 'isActive': True}
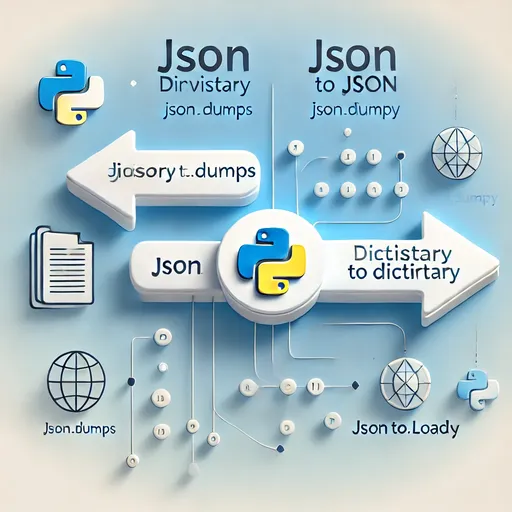
Conclusion
JSON and Python Dictionary have similar structures, but they differ in purpose and syntax. JSON is a standard format for data exchange, while Python Dictionary is suited for manipulating data within Python code. The json
module in Python makes it very easy to convert between JSON and Dictionary, so make sure to utilize it according to your needs!
Additional Tips
- When saving JSON files, using the
indent
option can help create files that are easier for humans to read. Always prioritize readability when dealing with data files. - Always keep in mind the difference between Boolean values and
null
/None
when converting between JSON and Dictionary.
There are no comments.