Django's sessions play a crucial role in maintaining user state. However, there are times when session data needs to be deleted for reasons such as security or performance. For this purpose, Django offers various methods related to session deletion (flush()
, pop()
, del
).
In this post, we will compare the functionality, features, use cases of each method, and when it is appropriate to use which method.
1. session.flush()
Definition
flush()
deletes all session data and creates a new unique session ID (cookie). Since the user authentication status is not maintained, it has the effect of logging the user out.
Features
- Total Initialization: Deletes all session data and generates a new session ID.
- Enhanced Security: Useful for completely resetting session information to ensure no session data remains when a user logs out.
Use Cases
- User Logout:
from django.contrib.auth import logout def user_logout(request): logout(request) request.session.flush() return redirect('login_page')
- Session Reset for Security Enhancement: Completely resets session data to prevent session hijacking or when suspicious activity is detected.
2. session.pop(key, default=None)
Definition
pop(key)
deletes the data for a specific key in the session and returns the value for that key. If the key does not exist, it returns the default
value.
Features
- Key-Based Deletion: Useful for partially deleting session data.
- Safe Deletion:
No error occurs if the key to be deleted does not exist; instead, it returns the
default
value.
Use Cases
- Remove Cart Item:
def remove_item(request, item_id): cart = request.session.get('cart', {}) removed_item = cart.pop(item_id, None) request.session['cart'] = cart return JsonResponse({'removed_item': removed_item})
- Delete Specific Data: Deletes only specific data such as cart items, form data, or one-time messages.
3. del request.session[key]
Definition
del
keyword deletes the data for a specific key in the session. If the key to be deleted does not exist, a KeyError
occurs.
Features
- Key-Based Deletion:
Similar to
pop()
, it deletes specific key data, but requires error handling. - Strict Deletion: The key to be deleted must exist, so try-except may be necessary for code stability.
Use Cases
- Manual Data Management:
def clear_session_data(request, key): try: del request.session[key] except KeyError: pass
- Forced Deletion: Used when specific data must be deleted.
4. Method Comparison
Method | Description | Use Case | Advantages | Disadvantages |
---|---|---|---|---|
session.flush() |
Deletes entire session and generates a new session ID | User logout, security enhancement | Prevents session hijacking, fully deletes data | Deletes all data, including authentication status |
session.pop() |
Deletes specific key and returns the value | Removing cart items, deleting one-time messages | No error if deletion key is missing | Need to check default if deletion fails |
del session[key] |
Deletes specific key | Forced deletion of specific data | Strict confirmation of key existence | Raises KeyError if key is absent |
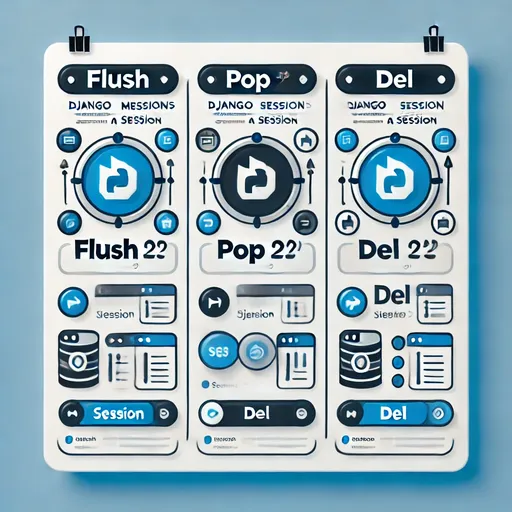
5. Precautions When Using
5.1 Security
- Using
flush()
: In applications where security is crucial, always useflush()
during logout to reset session data, preventing session hijacking. - Partial Data Deletion:
When deleting specific data, use
pop()
ordel
to avoid deleting unnecessary session data.
5.2 Performance
As session data grows, deletion operations can also affect performance. flush()
deletes the entire session, so caution is needed when handling large session data.
6. Selecting Methods Based on Scenarios
- Handling User Logout
def logout_view(request): request.session.flush() return redirect('login')
- Removing Cart Items
def remove_cart_item(request, item_id): cart = request.session.get('cart', {}) cart.pop(item_id, None) request.session['cart'] = cart return redirect('cart_page')
- Forced Deletion of Specific Data
def delete_user_setting(request, key): try: del request.session[key] except KeyError: pass
7. Summary
Situation | Recommended Method | Description |
---|---|---|
Deleting the entire session | flush() |
Deletes all data and generates a new session ID. |
Deleting only specific keys | pop() |
Deletes specific data safely. |
When the key must exist | del |
Force deletion of specific data. |
The session deletion methods in Django require appropriate choices based on the situation. Choose the suitable method to manage session data considering security and performance! 😊
There are no comments.