When writing code in Python, errors can occur for various reasons. These errors often cause the code to stop running, but Python allows for easy error handling using the try-except
statement. By utilizing this structure, the program can continue safely even if an error occurs in a specific statement.
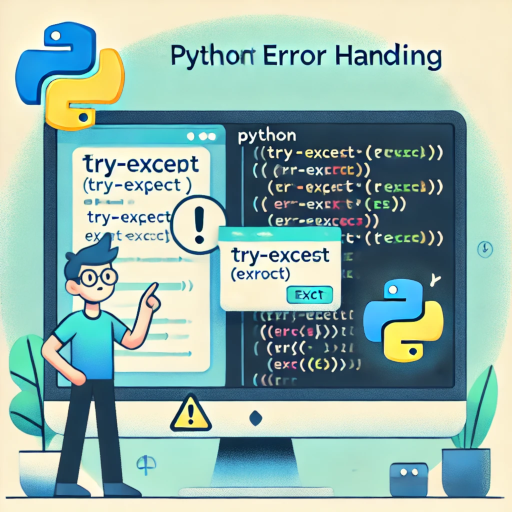
Understanding Python's try-except
statement equips you with the ability to identify and fix reasons for code failure. Below is a simple example of handling a ZeroDivisionError
.
try:
result = 10 / 0
except ZeroDivisionError:
result = "Cannot divide by zero"
print(result) # Cannot divide by zero
In the above code, when a division by zero error occurs, it prints the message "Cannot divide by zero" and allows the program to continue safely. Python is designed to enable beginners to handle errors easily, allowing them to freely experiment while writing code.
Comparison of Error Handling in C++ and Python
In another language like C++, exception handling is done using the try-catch
structure. However, after handling exceptions, you must specify the type precisely, and you must manage memory every time an error occurs, which can be cumbersome. For example, handling a division by zero situation in C++ should be written as follows.
#include <iostream>
int main() {
try {
int result = 10 / 0;
} catch (const std::exception& e) {
std::cout << "Error: " << e.what() << std::endl;
}
return 0;
}
In C++, while using the try-catch
structure, you need to detail the catch
block according to the type of error, and appropriately manage memory whenever an error occurs. In contrast, Python allows for easy error handling with a concise syntax without these complex processes. The try-except
construct in Python helps maintain the flow of the program even when an error occurs, favoring intuitive and straightforward code writing.
Advantages of the try-except Statement
- Program Stability: It prevents the code from stopping unexpectedly and ensures stable execution.
- Handling By Error Type: You can use multiple
except
clauses to handle specific errors appropriately. - Debugging and Problem Solving: It provides room to identify and rectify issues in the code.
Common Errors and How to Handle Them
There are various errors that can be handled using the try-except
structure in Python. Here are some errors frequently encountered by beginners and examples of their handling.
- ZeroDivisionError: This error occurs during division by zero and can be safely handled using
try-except
. - FileNotFoundError: This error occurs when trying to open a non-existent file, and it can be handled by notifying the user if the file is not available.
- ValueError: This occurs when an unexpected value is inputted and is particularly useful when a string is provided where a number is expected.
For instance, when opening a file, you can handle a FileNotFoundError
as follows.
try:
with open("non_existent_file.txt", "r") as file:
content = file.read()
except FileNotFoundError:
content = "File not found. Please check the file path."
print(content) # File not found. Please check the file path.
Tips for Using the try-except Statement
- Specify Errors Accurately: Instead of catching all errors, it's better to specify the expected types of errors.
- Log Error Messages: Rather than simply notifying the user of an error, logging it can be helpful for tracking down issues.
- Avoid Catching All Errors at Once: It's best to handle exceptions based on the most specific type of error possible.
Conclusion
The Python try-except
statement allows beginners to manage errors easily and run code safely. This construct prevents code interruptions and provides an environment for beginners to learn coding through free experimentation. Python continues to be beloved by many programmers, and it would be great for newcomers to learn and use these convenient features of Python more.
Add a New Comment