Data retrieval using Django ORM is one of the most powerful features of Django. QuerySet is the fundamental tool for efficiently fetching data from Django models, allowing for even complex data queries to be handled easily. In this article, we will explore what QuerySet is, how it can be utilized, and various features that are useful for data retrieval.
1. What is QuerySet?
QuerySet is a collection of objects used in Django ORM to retrieve or manipulate data from a database. Simply put, a QuerySet can be viewed as a set of results from executing a database query. Using QuerySet, you can fetch, filter, and manipulate model objects.
QuerySet encapsulates interactions with the database, allowing developers to query or manipulate data using intuitive Python code without writing raw SQL.
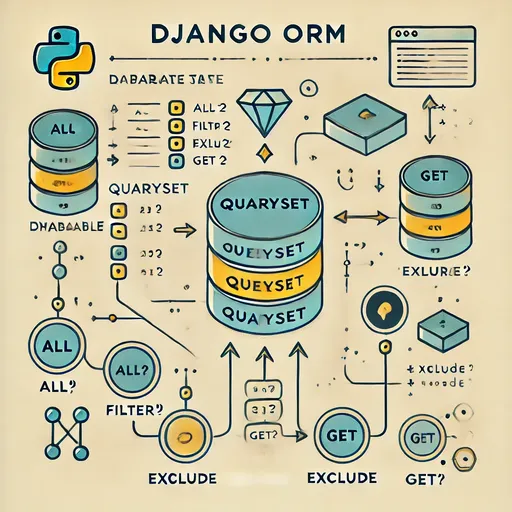
What you can do with QuerySet
- Data Retrieval: You can retrieve records stored in the database based on various conditions.
- Filtering: You can apply multiple conditions to filter out the data you want.
- Sorting and Slicing: You can sort data in the desired order or retrieve data within a specified range.
2. Types of Basic Retrieval Methods and Usage Examples
Django ORM provides various basic retrieval methods that are extremely useful for easily querying and filtering data.
2.1 all()
This method is used when you want to fetch all data of a model.
from myapp.models import Post
posts = Post.objects.all()
Post.objects.all()
retrieves all objects stored in the Post model.
2.2 filter()
This method is used to fetch only the objects that match specific conditions.
published_posts = Post.objects.filter(status='published')
filter()
queries objects that meet the conditions, and the return result is a QuerySet.
2.3 exclude()
This method is used to fetch objects excluding certain conditions.
draft_posts = Post.objects.exclude(status='published')
exclude()
queries objects that do not meet the conditions, in contrast tofilter()
.
2.4 get()
This method is used to fetch a single object that meets specific conditions. However, an error occurs if no matching object exists or if more than one exists.
post = Post.objects.get(id=1)
get()
is used when you want to query exactly one object; if there are no results or multiple results,DoesNotExist
orMultipleObjectsReturned
exceptions will be raised.
3. Operators and Filtering in ORM
QuerySet can handle complex filter conditions using various operators. These operators allow filtering data with very specific conditions.
3.1 exact
This operator is used to find exact matching values.
post = Post.objects.filter(title__exact='My First Post')
title__exact
filters objects whose title exactly matches'My First Post'
.
3.2 icontains
This operator is used to search for partial strings without case sensitivity.
posts = Post.objects.filter(title__icontains='django')
title__icontains
retrieves all posts whose title contains'django'
.
3.3 gt
, lt
(Greater than, Less than)
This is used to set conditions for values greater than or less than for numbers or date values.
recent_posts = Post.objects.filter(publish_date__gt='2024-01-01')
publish_date__gt
filters posts published after2024-01-01
.gt
means greater than, andlt
means less than.
In addition, various operators such as startswith
, endswith
, and in
can be used to implement a variety of filtering conditions easily.
4. Reverse Lookup: Querying Relationships Between Models Connected by Foreign Keys
In Django ORM, reverse lookup is a way to query relationships between models using foreign keys. Utilizing reverse lookup allows you to conveniently retrieve data about the parent-child relationship in relational databases.
4.1 Reverse Lookup Using related_name
When defining a foreign key, using the related_name
option allows you to use a more readable name during reverse lookup.
Example
class Post(models.Model):
title = models.CharField(max_length=100)
content = models.TextField()
class Comment(models.Model):
post = models.ForeignKey(Post, on_delete=models.CASCADE, related_name='comments')
text = models.TextField()
Usage
post = Post.objects.get(id=1)
comments = post.comments.all()
post.comments.all()
allows you to retrieve all comments associated with that post. If therelated_name
was not set, the defaultcomment_set
would be used as the name.
Conclusion
The QuerySet of Django ORM is a powerful tool for interacting with databases, enabling efficient querying and manipulation of desired data through various methods and operators. In this article, we explored the basic usage of QuerySet along with methods for filtering data and performing reverse lookups.
The aspects introduced above are just the very basics of ORM's retrieval features. In practice, Django's QuerySet offers even more diverse and powerful methods, and you can combine these methods to query data. In the future, I will write posts introducing and analyzing the various methods of QuerySet one by one.
In the next installment, we will discuss QuerySet performance optimization and the concept of lazy evaluation, to explore better approaches for data access. Stay tuned!
There are no comments.