In the previous post, I introduced how to handle data using Django's ORM, specifically the CRUD (Create, Read, Update, Delete) operations. This time, I will analyze more deeply what the objects
manager used in the examples is, how it is structured, and what it can do. Understanding objects
is crucial for grasping Django ORM properly.
1. What is the objects
manager?
The most commonly seen component in the basic structure of a Django model is the manager called objects
. objects
is called Django's model manager, and it serves the purpose of querying or manipulating database records. In simpler terms, the objects
manager acts as a bridge between the model class and the database.
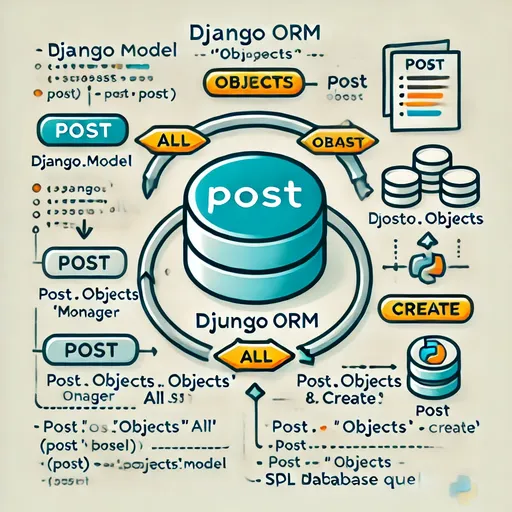
The basic property of the model class, objects
, is an instance of the Manager class provided by Django. Through this instance, it communicates with the database and performs actions such as creating, retrieving, updating, and deleting data. For instance, you can access the data using methods like Post.objects.all()
or Post.objects.create()
.
2. Role of the Manager class
The Manager class is the interface with the database that is automatically provided for Django models. It allows you to execute various queries. By default, Django automatically creates a Manager
named objects
for each model, which is used to handle most CRUD operations.
You may already have some knowledge or experience regarding how CRUD is implemented through objects
. The example code was covered in the previous post, so I will omit it here.
3. Creating a custom Manager
While the provided objects
manager is very useful, there are times when you need to reuse specific query logic or perform more complex queries. In such cases, you can create a custom manager to add the desired functionality.
3.1 Defining a custom Manager class
For example, let's create a custom manager that filters only the published posts.
from django.db import models
class PublishedManager(models.Manager):
def get_queryset(self):
return super().get_queryset().filter(status='published')
class Post(models.Model):
title = models.CharField(max_length=100)
content = models.TextField()
status = models.CharField(max_length=10, choices=[('draft', 'Draft'), ('published', 'Published')])
publish_date = models.DateTimeField(auto_now_add=True)
# Adding a custom manager in addition to the default objects manager
published = PublishedManager()
In the above example, we defined a custom manager called PublishedManager
and added it to the Post
model. This PublishedManager
filters and returns only the posts with a status of 'published'
. Now, calling Post.published.all()
will retrieve only the published posts.
3.2 Utilizing a custom manager
- Reducing duplicate code: Instead of adding filtering conditions repeatedly, using a custom manager can reduce code duplication.
- Encapsulating specialized query logic: You can customize your query logic to fit specific business requirements and manage it cleanly within the model.
4. Various operations possible with the objects
manager
The objects
manager in Django supports a variety of database operations beyond just the basic CRUD functionalities.
4.1 Data Filtering
filter()
: Retrieves multiple objects that match certain conditions.published_posts = Post.objects.filter(status='published')
exclude()
: Retrieves objects that do not match specific conditions.draft_posts = Post.objects.exclude(status='published')
4.2 Sorting and Slicing
- Sorting: You can sort data using the
order_by()
method.recent_posts = Post.objects.all().order_by('-publish_date')
- Slicing: Similar to Python's list slicing, you can slice a queryset to retrieve data within a specific range.
first_three_posts = Post.objects.all()[:3]
4.3 Using Aggregate Functions
The objects
manager also provides aggregate functions. For instance, if you want to know the number of posts, you can use the count()
method.
post_count = Post.objects.count()
Additionally, you can use the aggregate()
method to perform average, sum, and other aggregate operations.
from django.db.models import Avg
average_length = Post.objects.aggregate(Avg('content_length'))
5. How the objects
manager works
The objects
manager internally creates an object called a QuerySet. A queryset is a collection of queries to fetch data from the database. Each time methods like objects.all()
or objects.filter()
are called, Django generates a queryset and sends that query to the database to retrieve the results when necessary.
Querysets use lazy evaluation. This means that when a queryset is created, it does not actually access the database, but rather executes the database query at the point when the data is needed. This prevents unnecessary query executions and allows for efficient data processing.
Wrapping up this post
The objects
manager in Django simplifies interaction with the database and helps handle data more easily. Beyond the basic CRUD functionalities, you can define custom managers to reuse specific query logic or simply process more complex tasks. Furthermore, characteristics like lazy evaluation of querysets enhance efficient data handling.
By deeply understanding the objects
manager in Django ORM, you can manage databases more efficiently and write cleaner code while reducing duplication. In the future, increase the utility of model managers and consider incorporating custom managers whenever necessary. This will make complex data processing in web applications much easier and more flexible.
In the next post, I will discuss QuerySet and data retrieval in detail. One of the advantages of ORM is that "you don’t need to know SQL," so the content in the next post will be crucial to fully utilizing this advantage. Stay tuned for the next part.
Add a New Comment