If you've started web development and encountered the Django framework, you might have heard the term "ORM". Django ORM is one of the key tools that helps manage data easily in Django. In this post, we will explain the concept of Django ORM and how to use it in detail so that even beginners can understand.
1. What is ORM?
ORM stands for Object-Relational Mapping. In simple terms, think of it as a tool that helps you perform complex tasks related to databases easily with Python code.
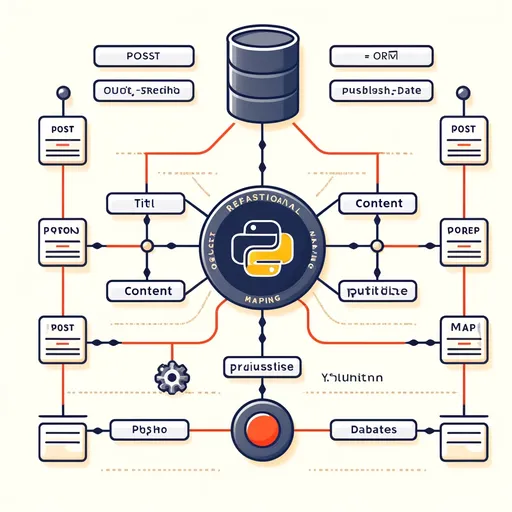
Typically, web applications need to perform tasks such as storing, retrieving, modifying, and deleting data in a database. However, databases are usually manipulated through a language called SQL. While SQL is a very useful language, it can be cumbersome for developers to write SQL queries manually each time, especially as the data becomes more complex and there are more relationships to manage.
ORM allows you to handle databases with a programming language like Python instead of writing SQL directly. With Django's ORM, you can manipulate the database using Python code without needing to know SQL queries. This is one of the biggest advantages of Django ORM.
2. Key Concepts of Django ORM
In Django, database tables are represented as models. A model is a class that defines the structure of the database and how to handle data, and Django ORM uses this model to automatically generate SQL queries and interact with the database.
2.1 Defining a Model
Models are defined as Python classes in Django. For example, let's create a simple model that can store blog posts.
from django.db import models
class Post(models.Model):
title = models.CharField(max_length=100)
content = models.TextField()
publish_date = models.DateTimeField(auto_now_add=True)
In the code above, we defined a model named Post
. This model has fields named title
, content
, and publish_date
. Each of these fields represents a column in the database, and this model will represent a table in the database.
title
: A string field that stores the title of the post, with a maximum length of 100 characters.content
: A field for storing the content of the post, which is a text field with no length limit.publish_date
: A date field that automatically records the date the post is published.
In this way, you can define the structure of a database table through a Python class. Django ORM will use this model to create the table and manage the data.
2.2 Database Operations
Once you've defined the model, let's perform some database operations using this model. The four basic operations are commonly referred to as CRUD: Create, Read, Update, Delete. Django ORM makes it easy to perform these CRUD operations.
2.2.1 Creating Data (Create)
If you want to save a post to the database, you can simply do it like this:
new_post = Post.objects.create(title='First Post', content='Write your post content here.')
Using the Post.objects.create()
method to create data adds a new record to the database.
2.2.2 Reading Data (Read)
If you want to retrieve data from the database, you can use methods like these:
all_posts = Post.objects.all() # Retrieve all posts
specific_post = Post.objects.get(id=1) # Retrieve the post with a specific id
Post.objects.all()
: Retrieves all posts from the database.Post.objects.get(id=1)
: Retrieves one piece of data that meets a specific condition, in this case, the post withid
1.
2.2.3 Updating Data (Update)
If you want to modify data, you first retrieve the data, then modify and save it.
specific_post = Post.objects.get(id=1)
specific_post.title = 'Updated Title'
specific_post.save() # Save the changes to the database
When you call the save()
method, the changes will be reflected in the database.
2.2.4 Deleting Data (Delete)
If you want to delete data, you can do it like this:
specific_post = Post.objects.get(id=1)
specific_post.delete() # Delete the specific post from the database
Calling the delete()
method will remove the corresponding record from the database.
3. Advantages of Django ORM
- No need to know SQL: Thanks to ORM, developers can handle data using Python code without needing to write complex SQL queries. This is a significant advantage, especially for beginners who may lack database knowledge.
- Database independence: Django ORM is compatible with various database engines such as MySQL, PostgreSQL, and SQLite. Therefore, after developing an application, if you need to switch databases, you can do so easily by changing the database settings without needing to modify the code.
- Increased productivity: Since you can handle data with Python code, the development speed increases, leading to higher productivity.
4. When to use ORM?
While ORM is convenient for interacting with databases, it may not be suitable for every situation. If a complex SQL query is needed, it can be more challenging to implement using ORM. In such cases, you might want to write SQL directly or use the Raw SQL feature provided by Django. However, for general CRUD operations or handling relational models, ORM is much more convenient and intuitive.
Conclusion
Django ORM is a powerful tool that simplifies interaction with databases. The ability to create, retrieve, modify, and delete data using Python code without writing SQL directly allows even beginners to manage data easily. If you intend to develop web applications with Django, understanding and utilizing the concept of ORM is important. This will enhance productivity and enable you to write more efficient code.
Hopefully, you now have a clearer understanding of what it means to define models and use ORM in Django. As you practice handling CRUD operations, you will appreciate the convenience of ORM even more.
There are no comments.