Python has a built-in function called hasattr
that allows you to easily check if an object has a specific attribute. This function is useful for checking whether an object has a property or method in object-oriented programming. In this post, we will explore how to use the hasattr
function and provide examples of how it can be utilized.
1. What is the hasattr
function?
The hasattr
function takes two arguments: object and attribute name (as a string). The function returns True
if the object has the specified attribute, and False
if it does not. This allows you to set up specific actions based on the presence or absence of an attribute.
- Syntax:
hasattr(object, "attribute_name")
- Return value:
- Returns
True
if the attribute exists on the object - Returns
False
if the attribute does not exist on the object
- Returns
2. Examples of Using the hasattr
Function
hasattr
is useful for performing conditional actions based on the existence of attributes. For instance, it can be used to call a specific method only if the object has that method.
Example 1: Checking Object Attributes
class Dog:
def __init__(self, name):
self.name = name
my_dog = Dog("Buddy")
# Check if the 'name' attribute exists
print(hasattr(my_dog, "name")) # Output: True
# Check if the 'age' attribute exists
print(hasattr(my_dog, "age")) # Output: False
In the above example, the my_dog
object has only the name
attribute defined. We are using hasattr
to check the existence of the name
and age
attributes.
Example 2: Conditional Execution Based on Method Existence
class Animal:
def sound(self):
print("Some sound")
class Dog(Animal):
def bark(self):
print("Woof!")
def make_sound(animal):
# Call 'bark' method if it exists on the animal
if hasattr(animal, "bark"):
animal.bark()
else:
animal.sound()
dog = Dog()
cat = Animal()
make_sound(dog) # Output: Woof!
make_sound(cat) # Output: Some sound
This example checks if the animal
object has the bark
method using hasattr
. If it does, bark()
is called; if not, the default sound()
method is called. This allows for flexible function behavior.
3. Differences Between hasattr
and getattr
While hasattr
checks for the existence of an attribute, getattr
is used to retrieve an attribute. getattr
can also be given a default value to return when the attribute does not exist.
Comparative Example:
class Person:
name = "Alice"
person = Person()
# hasattr: Check for attribute existence
print(hasattr(person, "name")) # Output: True
# getattr: Retrieve attribute value
print(getattr(person, "name", "Unknown")) # Output: Alice
# Default value returned when accessing a non-existing attribute with getattr
print(getattr(person, "age", "Not specified")) # Output: Not specified
hasattr
simply checks for presence, while getattr
can specify a default value, combining the functionalities of attribute retrieval and default value return.
4. Useful Applications of hasattr
- Dynamic Attribute Access: Useful in data models where attribute names are determined dynamically.
- Support for Polymorphic Behavior: When methods are not commonly defined across different classes,
hasattr
can check for the presence of a method and execute the appropriate action. - Flexible Exception Handling: Useful to prevent exceptions in cases where an attribute is absent.
The hasattr
function in Python makes it easy to check for the existence of attributes, enhancing code flexibility and helping to avoid exceptional situations. I have also experienced significant code clarity by using hasattr
in Django view functions. I needed to select between two different model objects based on specific conditions and send them to a template, where I had to reference the necessary values of the objects. By creating custom template filter tags and using hasattr
, I was able to keep the template code neat.
Try leveraging hasattr
in various scenarios to write object-oriented programming more flexibly and effectively!
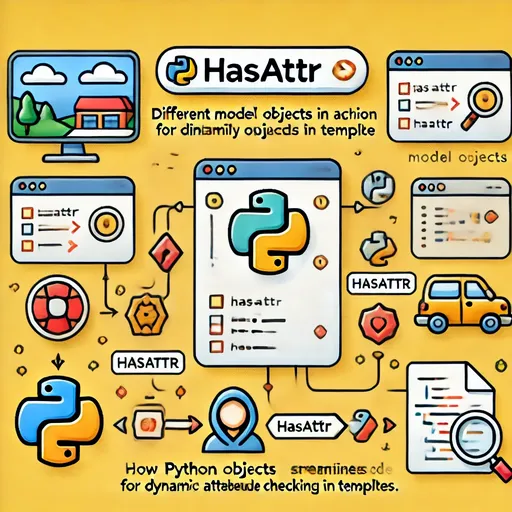
There are no comments.